Welcome to Code Tega! Today, we’re going to learn how to handle global state in React. We will do this by using the useContext
hook. This method is simple to set up, requires less code than other methods, and is a valuable tool that your clients and managers will appreciate. If you haven’t already, please subscribe as a way to support more tutorials and help the dev community. Thanks for watching, and let’s get started!
Managing State in React
In React, we commonly manage state using the useState
hook:
const [state, setState] = useState(initialState);
However, a common issue with useState
is that state can be reset when navigating between components. For instance, in a counter app, if the user navigates away and returns, the state resets to its initial value.
Introducing useContext
for Global State
To maintain state across different components, you can use a library like Redux, but I prefer using the useContext
hook due to its simplicity and reduced dependency on external libraries.
Setting Up useContext
Let’s create a context for our counter example.
1. Create a Context Folder in you src Directory:
- Create a folder named
context
. - Add a file named
CounterContext.jsx
.
2. Import Necessary Hooks:
import { createContext, useContext, useState, useEffect } from "react";
createContext
: Creates a context that components can provide or read.useContext
: Allows components to read and subscribe to context.useState
: Adds a state variable to your component.useEffect
: (Optional) Sycronizes a component with an external system
3. Create and Export Context:
const CountContext = createContext();
export function CountContextProvider({ children }) {
const [count, setCount] = useState(0);
return (
<CountContext.Provider value={{ count, setCount }}>
{children}
</CountContext.Provider>
);
}
export const UserCount = () => {
return useContext(CountContext);
};
4. Wrap Your App with the Context Provider:
In your index.js
file:
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
import { BrowserRouter } from "react-router-dom";
import { CountContextProvider } from './context/CounterContext';
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<BrowserRouter>
<CountContextProvider>
<App />
</CountContextProvider>
</BrowserRouter>
);
Use Context in Components:
In your Counter
component:
import React, { useState } from "react";
import { UserCount } from "./context/CounterContext";
export default function Counter() {
const { count, setCount } = UserCount();
const handleAddOne = () => {
setCount(count + 1);
};
return (
<div className="centerText">
<h1>Counter App</h1>
<h2>{count}</h2>
<button onClick={handleAddOne}>Add One</button>
</div>
);
}
Ensuring State Persistence on Navigation
To ensure that your counter retains its value when navigating, use <Link> components from react-router-dom
for navigation instead of using <a>
tags. As <a> tags will refresh the page on each navigation pass and re-render the state of your application
(Optional) Managing State Across Page Refreshes
For state persistence even on page refresh, you can use localStorage
:
1. Set Item in Component:
const handleAddOne = () => {
setCount(count + 1);
localStorage.setItem("count", count + 1);
};
2. Get Item in Context:
useEffect(() => {
const savedCount = localStorage.getItem('count');
if (savedCount) {
setCount(parseInt(savedCount, 10));
}
}, []);
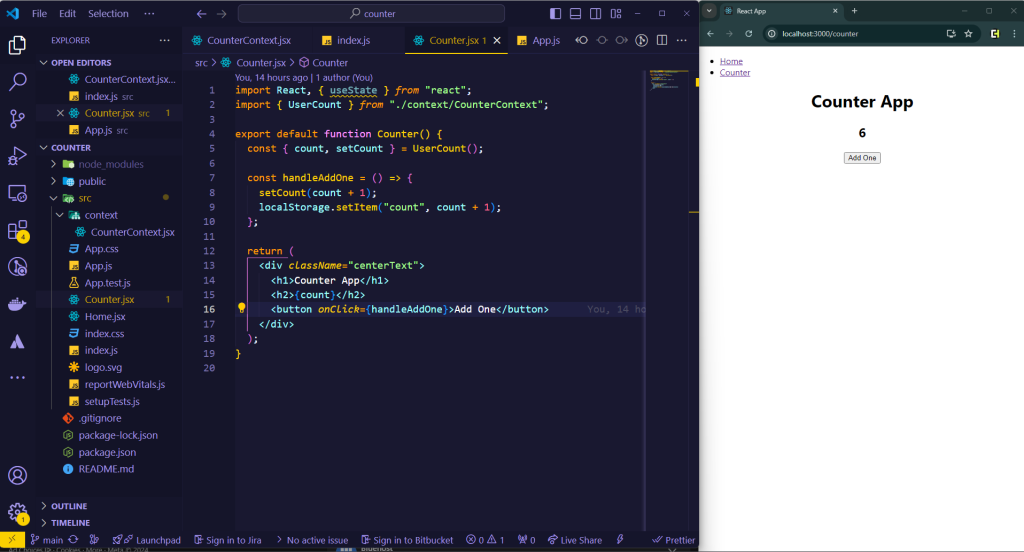
Conclusion
You’ve successfully set up global state using useContext
and learned how to handle global state in React! For navigation, ensure you’re using react-router-dom
and consider localStorage
for state persistence across page refreshes. Combining both methods provides a robust solution for managing global state.
If you found this tutorial helpful, please let me know what else you’d like to learn. Don’t forget to subscribe for more content. Good luck, and see you next time!
If you haven’t already checkout How To Create a Desktop App
Project Repo: https://github.com/CodeTega/contextCounter.git